13 min to read
Understanding xargs - Powerful Command-Line Utility for Argument Processing
Learn how to use xargs command effectively with practical examples
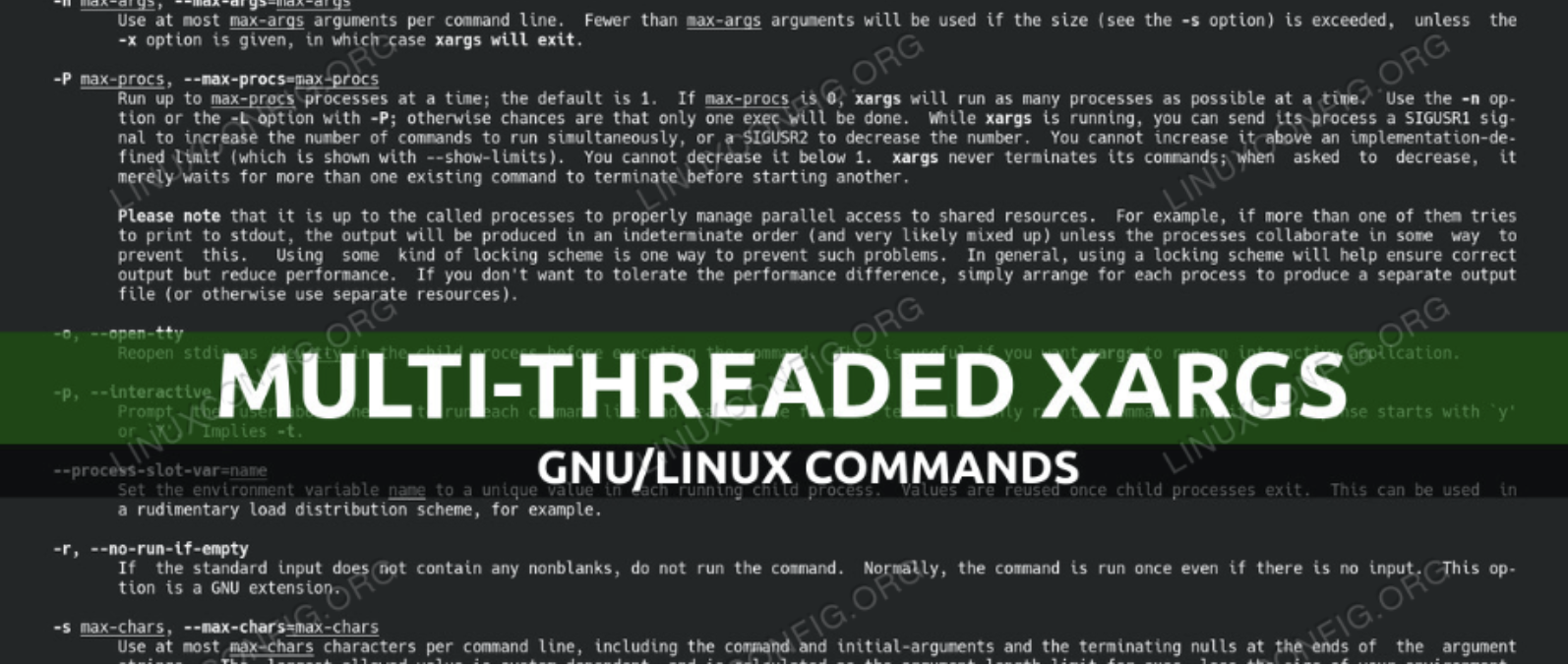
What is xargs?
It efficiently bridges commands together, allowing you to build complex command pipelines by converting standard input into command arguments.
Why is xargs so useful?
✅ Overcomes command line length limitations: Processes large input sets that would exceed command line limits
✅ Efficiency: Reduces the number of command invocations, improving performance
✅ Parallelism: Supports parallel processing to speed up operations
✅ Flexibility: Works with virtually any command that accepts arguments
✅ Pipeline integration: Seamlessly connects with other Unix/Linux tools
How xargs Works
Basic Syntax
command1 | xargs [options] [command2]
This tells xargs to take the output from command1 and use it as arguments for command2.
Default Behavior
If no command is specified, xargs defaults to using /bin/echo
:
echo "a b c" | xargs
# Output: a b c
Core Concept: Argument Handling
xargs reads items from standard input, delimited by blanks (spaces, tabs, newlines) and executes the command with those items as arguments:
echo "file1 file2 file3" | xargs ls -l
# Equivalent to: ls -l file1 file2 file3
Basic Examples
1. Working with find
Finding and processing files is one of the most common uses of xargs:
# Find all .log files and delete them
find /var/log -name "*.log" | xargs rm -f
# Find all .txt files and count their lines
find . -name "*.txt" | xargs wc -l
2. Transforming Standard Input
# Convert space-separated list to one item per line
echo "apple banana cherry" | xargs -n 1
# Output:
# apple
# banana
# cherry
# Add a prefix to each word
echo "file1 file2 file3" | xargs -n 1 echo "Processing:"
# Output:
# Processing: file1
# Processing: file2
# Processing: file3
3. Creating File Backups
# Find all .conf files and create a backup of each
find /etc -name "*.conf" | xargs -I{} cp {} {}.bak
4. Processing Output from Other Commands
# Get all running processes for user 'somaz' and display details
ps aux | grep somaz | grep -v grep | awk '{print $2}' | xargs ps -f
Essential Options
Option | Description | Example |
---|---|---|
-n |
Specify number of arguments per command | echo "1 2 3 4" | xargs -n 2 echo Output: 1 2 3 4 |
-I {} |
Replace string for each input item | echo "file1 file2" | xargs -I{} mv {} {}.bak Renames files to file1.bak and file2.bak |
-0 |
Input items are terminated by null, not whitespace | find . -name "*.txt" -print0 | xargs -0 rm Safely handles filenames with spaces |
-P |
Run up to max-procs processes at a time | find . -name "*.jpg" | xargs -P4 -I{} convert {} {}.png Processes 4 images simultaneously |
-t |
Print the command before executing | echo "file1 file2" | xargs -t rm Prints: rm file1 file2 |
-p |
Prompt user before execution | echo "file1 file2" | xargs -p rm Asks for confirmation |
Advanced Usage
Handling Filenames with Spaces and Special Characters
One common challenge is dealing with filenames that contain spaces or special characters:
# This approach will fail with spaces in filenames
find . -name "*.txt" | xargs rm # WRONG for files with spaces!
# Correct approach using -print0 and -0
find . -name "*.txt" -print0 | xargs -0 rm
The -print0
option of find outputs null-terminated strings, and the -0
option of xargs reads null-terminated strings.
Using Replacement Strings with -I
The -I
option allows you to specify a replacement string:
# Copy all .jpg files to /backup with the same names
find . -name "*.jpg" | xargs -I{} cp {} /backup/{}
# Create a directory for each .log file and move the file into it
find . -name "*.log" | xargs -I{} bash -c 'mkdir -p {}.dir && mv {} {}.dir/'
Executing Commands with Multiple Arguments
# Generate a list of files with different extensions and check if they exist
echo "file1.txt file2.pdf file3.doc" | xargs -n 1 ls -l 2>/dev/null || echo "File not found"
Parallel Processing with xargs
One of xargs’ most powerful features is its ability to run commands in parallel using the -P
option, which specifies the maximum number of processes to run simultaneously.
Sequential vs Parallel Execution
Sequential Execution (Default)
time for i in $(seq 1 5); do echo $[$RANDOM % 5 + 1]; done | xargs -I{} echo "sleep {}; echo 'Done! {}'" | xargs -I{} bash -c "{}"
# Sample output:
Done! 4
Done! 3
Done! 4
Done! 2
Done! 3
real 0m16.034s
user 0m0.033s
sys 0m0.005s
Parallel Execution (using -P option)
time for i in $(seq 1 5); do echo $[$RANDOM % 5 + 1]; done | xargs -I{} echo "sleep {}; echo 'Done! {}'" | xargs -P5 -I{} bash -c "{}"
# Sample output:
Done! 1
Done! 1
Done! 2
Done! 4
Done! 4
real 0m4.007s
user 0m0.016s
sys 0m0.003s
Performance Comparison:
- Sequential: ~16 seconds
- Parallel: ~4 seconds (75% time reduction)
Real-World Parallel Processing Examples
Parallel Image Processing
# Resize all JPG images to 800x600 using 4 parallel processes
find . -name "*.jpg" | xargs -P4 -I{} convert {} -resize 800x600 {}.resized.jpg
Parallel File Compression
# Compress multiple log files in parallel
find /var/log -name "*.log" | xargs -P8 -I{} gzip {}
Parallel HTTP Requests
# Fetch multiple URLs in parallel
cat urls.txt | xargs -P10 -I{} curl -s {} > /dev/null
While parallel processing can dramatically improve performance, it comes with some considerations:
- High -P values can overwhelm system resources (CPU, memory, I/O)
- Output from parallel processes may be interleaved and difficult to read
- Some commands may not be thread-safe
- Use process monitoring (like top or htop) to ensure system stability
Practical Use Cases
Kubernetes Resource Management
# Delete all pods in Error state
kubectl get po -n <namespace> | grep Error | awk '{print $1}' | xargs kubectl delete po -n <namespace>
# Delete all pods in Evicted state
kubectl get po -n <namespace> | grep Evicted | awk '{print $1}' | xargs kubectl delete po -n <namespace>
# Delete all pods in CrashLoopBackOff state
kubectl get po -n <namespace> | grep CrashLoopBackOff | awk '{print $1}' | xargs kubectl delete po -n <namespace>
Docker Container Management
# Remove all exited containers
sudo docker ps -a | grep Exit | cut -d ' ' -f 1 | xargs sudo docker rm
# Stop all running containers
docker ps -q | xargs docker stop
# Remove all dangling images
docker images -f "dangling=true" -q | xargs docker rmi
System Administration
# Find and delete all files older than 30 days
find /tmp -type f -mtime +30 | xargs rm -f
# Find large files and calculate their total size
find / -type f -size +100M | xargs du -ch | grep total$
# Kill all processes for a specific user
ps -u username | grep -v PID | awk '{print $1}' | xargs kill
Text Processing
# Find all Python files containing "TODO" and list them
grep -l "TODO" $(find . -name "*.py") | xargs wc -l
# Replace a string in multiple files
grep -l "old_text" *.txt | xargs sed -i 's/old_text/new_text/g'
Common Mistakes and Troubleshooting
Mistake 1: Not Handling Spaces in Filenames
# This will break with spaces in filenames
find . -name "*.txt" | xargs rm # WRONG!
# Correct approach
find . -name "*.txt" -print0 | xargs -0 rm
Mistake 2: Using -I{} Without a Command
# This doesn't work as expected
echo "file1 file2" | xargs -I{} # WRONG!
# Correct approach
echo "file1 file2" | xargs -I{} echo "Processing {}"
Mistake 3: Not Considering Command Line Limitations
Even with xargs, there are still maximum command line length limits:
# This might exceed command line length limits with thousands of files
find / -type f | xargs grep "pattern" # May fail with too many files
# Better approach
find / -type f -exec grep "pattern" {} \; # Or
find / -type f | xargs -n 100 grep "pattern" # Process in batches
Use these approaches to debug xargs commands:
- Add the -t option to print commands before execution:
xargs -t
- Use -p to prompt before execution:
xargs -p
- Test with echo first:
xargs echo
instead of the actual command - Start with a small subset of data to verify behavior
Alternatives to xargs
While xargs is powerful, there are situations where alternatives might be more appropriate:
Alternative | Description | When to Use |
---|---|---|
find -exec |
Execute commands directly from find | When working exclusively with find; when precise control over execution is needed |
parallel |
GNU Parallel - advanced parallel command execution | For complex parallel processing needs; better output control; more sophisticated job distribution |
Shell loops | Using for/while loops in shell scripts | When more complex control flow is needed; when processing needs to be more conditional |
awk |
Text processing language | When extensive text manipulation is needed before command execution |
Comparison Example: Finding and Processing Files
# Using xargs
find . -name "*.log" | xargs grep "error"
# Using find -exec
find . -name "*.log" -exec grep "error" {} \;
# Using a shell loop
for file in $(find . -name "*.log"); do
grep "error" "$file"
done
# Using GNU Parallel
find . -name "*.log" | parallel grep "error" {}
Summary
- xargs transforms standard input into command arguments, bridging commands together in powerful ways
- It overcomes command line length limitations by processing input in manageable chunks
- The -P option enables parallel processing, dramatically improving performance for suitable tasks
- For filenames with spaces or special characters, always use find -print0 with xargs -0
- The -I option provides flexible argument placement for commands
Comments