3 min to read
What is Linux Kernel?
A comprehensive guide to the Linux Kernel architecture and functionality
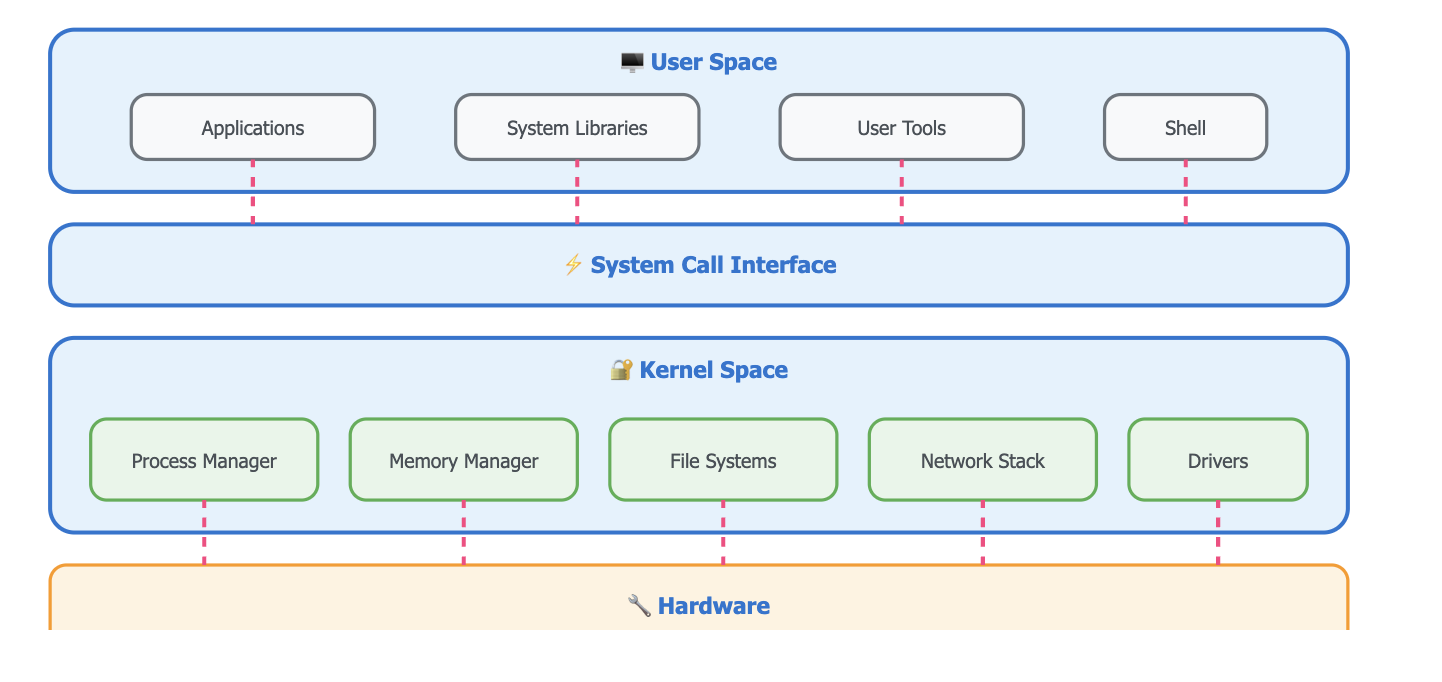
Overview
Let’s explore the Linux Kernel, the core component of the Linux operating system that manages hardware resources and provides essential services to system processes.
What is the Linux Kernel?
The kernel is the core part of any operating system. It’s the layer that communicates directly with hardware and provides essential services to applications and system processes.
Linux specifically uses a “monolithic” kernel design, meaning most core system functions (file system management, device drivers, memory management, etc.) are included within a single large binary.
🔄 Linux Kernel Architecture:
Main Responsibilities
Process Management → Controls process creation, scheduling, and termination
Memory Management → Handles virtual memory, paging, and memory allocation
Device Management → Manages hardware through device drivers
File System Management → Controls file storage and organization
Network Stack → Manages network communications and protocols
Key Components
1️⃣ System Call Interface
# Example of a system call in C
#include <stdio.h>
#include <unistd.h>
int main() {
pid_t pid = fork(); // fork() is a system call
if (pid == 0) {
printf("Child process\n");
} else {
printf("Parent process\n");
}
return 0;
}
2️⃣ Process Management
# View running processes
ps aux
# Check process priority
nice -n 10 ./my_program
# View process tree
pstree
3️⃣ Memory Management
# View memory usage
free -h
# Check virtual memory statistics
vmstat 1
# View detailed memory info
cat /proc/meminfo
Kernel Space vs User Space
Kernel Space
→ Privileged mode with full hardware access
→ Runs kernel code and device drivers
→ Manages system resources
User Space
→ Restricted mode for applications
→ Limited access to hardware
→ Must use system calls to request kernel services
Kernel Architecture Comparison
Feature | Monolithic Kernel | Micro kernel |
---|---|---|
Design | All services in kernel space | Minimal kernel, services in user space |
Performance | Faster (direct calls) | Slower (IPC overhead) |
Reliability | Less isolated | More isolated |
Size | Larger | Smaller |
Examples | Linux, Unix | MINIX, QNX |
Advanced Features
1️⃣ Kernel Modules
# List loaded modules
lsmod
# Load a module
sudo modprobe bluetooth
# Remove a module
sudo rmmod bluetooth
# Module information
modinfo bluetooth
2️⃣ System Control
# View kernel parameters
sysctl -a
# Modify kernel parameter
sudo sysctl -w vm.swappiness=60
# Load kernel parameter changes
sudo sysctl -p
Security Features
- SELinux
- AppArmor
- Capabilities
2. Memory Protection
- Address Space Layout Randomization (ASLR)
- Stack protection
- Memory page protection
3. Network Security
- Netfilter framework
- IPtables
- Network namespaces
Comments