11 min to read
Kubernetes Headless Services
Direct pod communication for stateful applications without load balancing
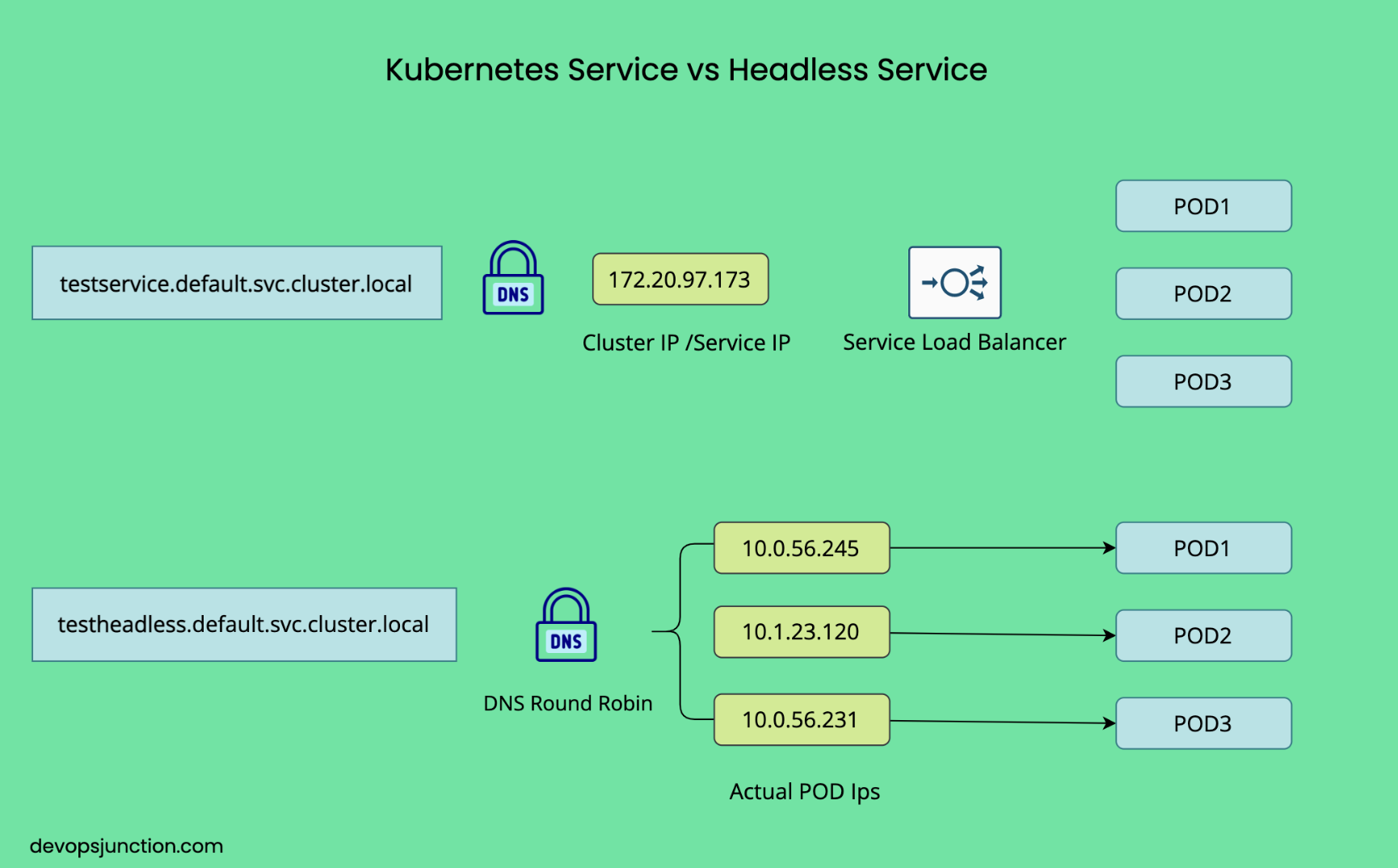
Overview
Kubernetes Headless Services provide direct pod access without load balancing or cluster IP assignment, making them ideal for stateful applications and databases. They solve the specific problem of providing stable networking identity to pods where direct communication is required.
Kubernetes offers several service types for different networking requirements:
- ClusterIP: Default type that provides internal load balancing with a stable virtual IP
- NodePort: Exposes the service on each node's IP at a static port
- LoadBalancer: Exposes the service externally using a cloud provider's load balancer
- Headless: Provides direct pod access with DNS-based discovery and no load balancing
Key Features and Concepts
No Cluster IP
Headless services are created by setting spec.clusterIP: None
in the service definition. This tells Kubernetes not to assign a virtual IP to the service and instead to handle DNS resolution differently.
apiVersion: v1
kind: Service
metadata:
name: headless-service
spec:
clusterIP: None # This is what makes it "headless"
selector:
app: my-app
ports:
- port: 80
targetPort: 8080
DNS Records for Pods
Unlike regular services that provide a single DNS entry pointing to a virtual IP, headless services create DNS records for each individual pod:
DNS Record | Resolution |
---|---|
my-service.default.svc.cluster.local |
Multiple A records (one for each pod IP) |
pod-0.my-service.default.svc.cluster.local |
IP address of pod-0 |
pod-1.my-service.default.svc.cluster.local |
IP address of pod-1 |
pod-2.my-service.default.svc.cluster.local |
IP address of pod-2 |
Direct Pod Communication
Headless services allow clients to connect directly to specific pods, bypassing the service abstraction layer that typically handles load balancing:
Use Cases for Headless Services
StatefulSet Integration
Headless services are commonly paired with StatefulSets to provide stable network identities for pods. Each pod in a StatefulSet gets a predictable name and DNS entry.
apiVersion: apps/v1
kind: StatefulSet
metadata:
name: web
spec:
serviceName: "headless-service" # References the headless service
replicas: 3
selector:
matchLabels:
app: web
template:
metadata:
labels:
app: web
spec:
containers:
- name: nginx
image: nginx:1.20
ports:
- containerPort: 80
When this StatefulSet is created with the headless service, it generates pods with the following DNS names:
web-0.headless-service.default.svc.cluster.local
web-1.headless-service.default.svc.cluster.local
web-2.headless-service.default.svc.cluster.local
Databases and Distributed Systems
Headless services are ideal for database clusters and distributed systems that need direct pod-to-pod communication:
- Cassandra: Nodes need to communicate directly with each other for gossip protocol
- Elasticsearch: Cluster formation requires direct node-to-node communication
- Kafka: Brokers need stable, predictable DNS names for replication
- MongoDB: Replica sets need to identify primary and secondary instances
- ZooKeeper: Ensemble members need direct access to each other
Example with Cassandra:
apiVersion: v1
kind: Service
metadata:
name: cassandra
labels:
app: cassandra
spec:
clusterIP: None
selector:
app: cassandra
ports:
- port: 9042
name: cql
- port: 7000
name: intra-node
- port: 7001
name: tls-intra-node
DNS Resolution and Behavior
A/AAAA DNS Query
When you perform a DNS query against a headless service, the response contains multiple A records - one for each pod backing the service:
$ nslookup cassandra.default.svc.cluster.local
Name: cassandra.default.svc.cluster.local
Address: 10.244.2.6
Address: 10.244.1.8
Address: 10.244.3.9
SRV Records
For more detailed service discovery, Kubernetes also creates SRV records for headless services:
$ dig SRV cassandra.default.svc.cluster.local
;; ANSWER SECTION:
cassandra.default.svc.cluster.local. 30 IN SRV 0 50 9042 cassandra-0.cassandra.default.svc.cluster.local.
cassandra.default.svc.cluster.local. 30 IN SRV 0 50 9042 cassandra-1.cassandra.default.svc.cluster.local.
cassandra.default.svc.cluster.local. 30 IN SRV 0 50 9042 cassandra-2.cassandra.default.svc.cluster.local.
SRV records include additional information beyond just IP addresses:
- Priority: Lower values are preferred (0 in this example)
- Weight: Relative weight for entries with the same priority (50 here)
- Port: The port where the service is found (9042)
- Target: The hostname of the target machine
Client-Side Load Balancing
Request Distribution
With headless services, traffic distribution is handled by the client rather than Kubernetes:
Client Behavior | Description | Example Client |
---|---|---|
Random Selection | Client picks one IP randomly from DNS results | Simple HTTP clients, some database drivers |
First IP Only | Client always uses the first IP in the results | Basic implementations with no load balancing |
Round Robin | Client cycles through all IPs in the results | Advanced clients, custom implementations |
Smart Routing | Client routes based on custom logic | Service mesh proxies, database connection pools |
DNS Caching Considerations
DNS caching can significantly impact how clients interact with headless services:
Most clients and DNS resolvers cache results
↓
Pod IPs may change during scaling or pod replacement
↓
Cached DNS records can point to outdated pod IPs
↓
Solutions:
- Set appropriate TTL values
- Implement client-side retry logic
- Consider service mesh for advanced service discovery
Implementation Examples
Basic Headless Service
apiVersion: v1
kind: Service
metadata:
name: headless-service
spec:
clusterIP: None
selector:
app: my-app
ports:
- protocol: TCP
port: 80
targetPort: 8080
Headless Service with External Name
For cases where you need to reference an external service without proxying:
apiVersion: v1
kind: Service
metadata:
name: external-db
spec:
type: ExternalName
clusterIP: None # Can be omitted as ExternalName services don't use clusterIP
externalName: database.example.com
Headless Service with Manual Endpoints
For scenarios where you need to manually specify the endpoints:
apiVersion: v1
kind: Service
metadata:
name: manual-endpoints
spec:
clusterIP: None
ports:
- protocol: TCP
port: 80
targetPort: 8080
---
apiVersion: v1
kind: Endpoints
metadata:
name: manual-endpoints # Must match service name
subsets:
- addresses:
- ip: 192.168.1.11
- ip: 192.168.1.12
- ip: 192.168.1.13
ports:
- port: 8080
protocol: TCP
Headless vs Regular Services
clusterIP: None] C -->|No| E[ClusterIP Service] C -->|Yes| F{Cloud Provider?} F -->|Yes| G[LoadBalancer Service] F -->|No| H[NodePort Service
or Ingress] style D fill:#bbf,stroke:#333,stroke-width:2px
Detailed Comparison
Feature | Headless Service | Regular Service |
---|---|---|
ClusterIP | None | Assigned |
Load Balancing | No (client-side only) | Yes (kube-proxy handles) |
DNS Records | Per-pod A/AAAA records | Single A/AAAA record for service |
Direct Pod Access | Yes | No (routed through virtual IP) |
Stable Pod Identity | Yes (with StatefulSet) | No (pods are anonymous) |
Use Case | Stateful apps, database clusters | Stateless apps, microservices |
Client Complexity | Higher (must handle multiple IPs) | Lower (single IP, proxied access) |
Session Affinity | Client controlled | Can be configured in service |
Best Practices and Considerations
When to Use Headless Services
- Direct pod-to-pod communication
- Stable, predictable DNS names for pods
- Client-side load balancing or custom routing logic
- Support for applications that manage their own service discovery
- Zero overhead in the connection path
When to Avoid Headless Services
- Simple load balancing is required
- Client doesn't need to be aware of individual pods
- You need session affinity managed by Kubernetes
- Applications can't handle DNS-based service discovery
- You need consistent distribution of traffic
Testing Headless Services
To verify your headless service is working correctly:
# Check the service | |
kubectl get svc headless-service | |
# Look for endpoints | |
kubectl get endpoints headless-service | |
# Test DNS resolution | |
kubectl run -it --rm --restart=Never debug --image=curlimages/curl -- \ | |
sh -c "nslookup headless-service.default.svc.cluster.local" | |
# Try accessing individual pods (if part of StatefulSet) | |
kubectl run -it --rm --restart=Never debug --image=curlimages/curl -- \ | |
sh -c "curl pod-0.headless-service.default.svc.cluster.local:80" |
Advanced Scenarios
Service Mesh Integration
Service meshes like Istio can enhance headless services with:
- Advanced traffic control
- Mutual TLS authentication
- Detailed metrics and monitoring
- Enhanced service discovery
apiVersion: networking.istio.io/v1beta1
kind: DestinationRule
metadata:
name: cassandra-destination
spec:
host: cassandra.default.svc.cluster.local
trafficPolicy:
loadBalancer:
simple: ROUND_ROBIN
connectionPool:
tcp:
maxConnections: 100
outlierDetection:
consecutive5xxErrors: 3
interval: 30s
baseEjectionTime: 30s
Custom DNS Policies
For applications with specific DNS resolution requirements:
apiVersion: v1
kind: Pod
metadata:
name: custom-dns-pod
spec:
dnsPolicy: "None"
dnsConfig:
nameservers:
- 1.2.3.4
searches:
- ns1.svc.cluster.local
- my.dns.search.suffix
options:
- name: ndots
value: "2"
containers:
- name: dns-example
image: nginx
Key Points
-
Definition
- Services with clusterIP: None
- No load balancing
- DNS returns pod IPs directly -
Primary Benefits
- Stable network identity
- Direct pod communication
- Ideal for stateful applications -
Implementation
- Pair with StatefulSets
- Requires DNS-aware clients
- Client handles connection management
Comments