5 min to read
Kubernetes Deployment Strategies
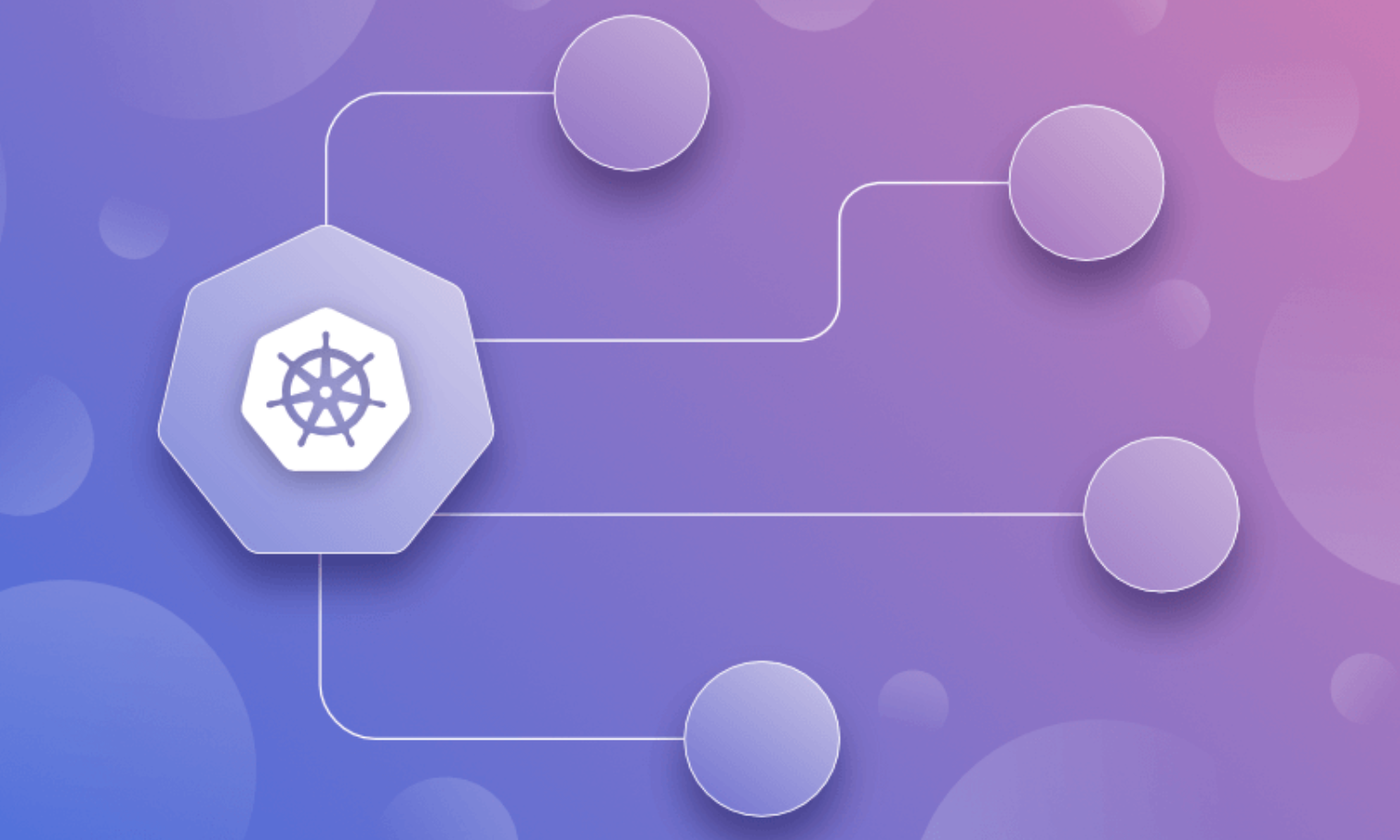
Overview
Kubernetes deployment strategies define how to update Pods or sets of Pods when deploying new application versions or container images.
- RollingUpdate is the default strategy.
- Recreate is the strategy that terminates all existing pods before creating new ones.
🔄 Basic Deployment Strategies
1. RollingUpdate (Default)
spec:
strategy:
type: RollingUpdate
rollingUpdate:
maxUnavailable: 1 # Maximum number of pods that can be unavailable during update
maxSurge: 1 # Maximum number of pods that can be created above desired number
- Gradually updates pods in small batches
- Minimizes downtime during updates
- Ideal for maintaining high availability
- Controls update pace with maxUnavailable and maxSurge parameters
2. Recreate
spec:
strategy:
type: Recreate
- Terminates all existing pods before creating new ones
- Results in downtime between versions
- Ensures clean deployment of new version
- Suitable for development environments or when downtime is acceptable
Strategy Comparison Table
Strategy | Downtime | Resource Usage | Complexity | Rollback |
---|---|---|---|---|
RollingUpdate | Minimal | Normal | Low | Gradual |
Recreate | Yes | Minimal | Very Low | Immediate |
Blue/Green | None | Double | High | Immediate |
Canary | None | Variable | High | Simple |
Advanced Deployment Strategies
1. Blue/Green Deployment
- Maintains two identical environments (blue and green)
- Switches traffic instantly between versions
- Provides zero downtime deployment
- Enables immediate rollback capability
- Requires double the resources
# Blue/Green Deployment Service
apiVersion: v1
kind: Service
metadata:
name: my-app
spec:
selector:
app: my-app
version: blue # Switch between blue and green
ports:
- protocol: TCP
port: 80
targetPort: 8080
2. Canary Deployment
- Gradually rolls out changes to a subset of users
- Enables risk mitigation through partial deployment
- Allows testing with real user traffic
- Requires sophisticated traffic control
# Canary Deployment with Ingress
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
annotations:
nginx.ingress.kubernetes.io/canary: "true"
nginx.ingress.kubernetes.io/canary-weight: "20"
Strategy Selection Guide
-
RollingUpdate
- Need continuous availability
- Can handle both versions simultaneously
- Have limited resources -
Recreate
- Need clean slate deployment
- Can tolerate downtime
- Have version incompatibility issues -
Blue/Green
- Need zero downtime
- Have sufficient resources
- Need immediate rollback capability -
Canary
- Need gradual validation
- Want to test with real users
- Can manage complex traffic routing
🔍 Deployment Health Checks
1. Readiness Probe
spec:
containers:
- name: my-app
readinessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 5
periodSeconds: 10
- Determines when a pod is ready to accept traffic
- Essential for smooth rolling updates
- Prevents routing to partially started pods
2. Liveness Probe
spec:
containers:
- name: my-app
livenessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 15
periodSeconds: 20
- Checks if a pod is healthy and running
- Automatically restarts unhealthy pods
- Crucial for maintaining application reliability
Advanced Configuration Options
1. Progressive Delivery with Argo Rollouts
apiVersion: argoproj.io/v1alpha1
kind: Rollout
metadata:
name: my-app-rollout
spec:
strategy:
canary:
steps:
- setWeight: 20
- pause: {duration: 1h}
- setWeight: 40
- pause: {duration: 1h}
- Automated canary analysis
- Traffic weight management
- Metric-based promotion/rollback
2. A/B Testing Configuration
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
annotations:
nginx.ingress.kubernetes.io/canary: "true"
nginx.ingress.kubernetes.io/canary-by-header: "User-Country"
nginx.ingress.kubernetes.io/canary-by-header-value: "US"
- Feature testing with specific user segments
- Header-based routing
- Geographical distribution
Common Pitfalls and Best Practices
Common Issues to Avoid:
- Not setting appropriate resource limits and requests
- Ignoring readiness/liveness probe configuration
- Insufficient monitoring during deployments
- Not planning for rollback scenarios
Best Practices:
- Always use version tags instead of 'latest'
- Implement proper health checks
- Monitor deployment metrics
- Maintain deployment history for rollbacks
- Use pod disruption budgets for high availability
Monitoring Deployments
Key Metrics to Monitor
- Deployment success rate
- Rollout duration
- Error rates during deployment
- Resource utilization
- Response times
- Pod health status
Example Prometheus Query
rate(http_requests_total{status!~"5.."}[5m])
/
rate(http_requests_total[5m])
Comments