4 min to read
Understanding Helm Chart Template Syntax
A comprehensive guide to Helm Chart templating and best practices
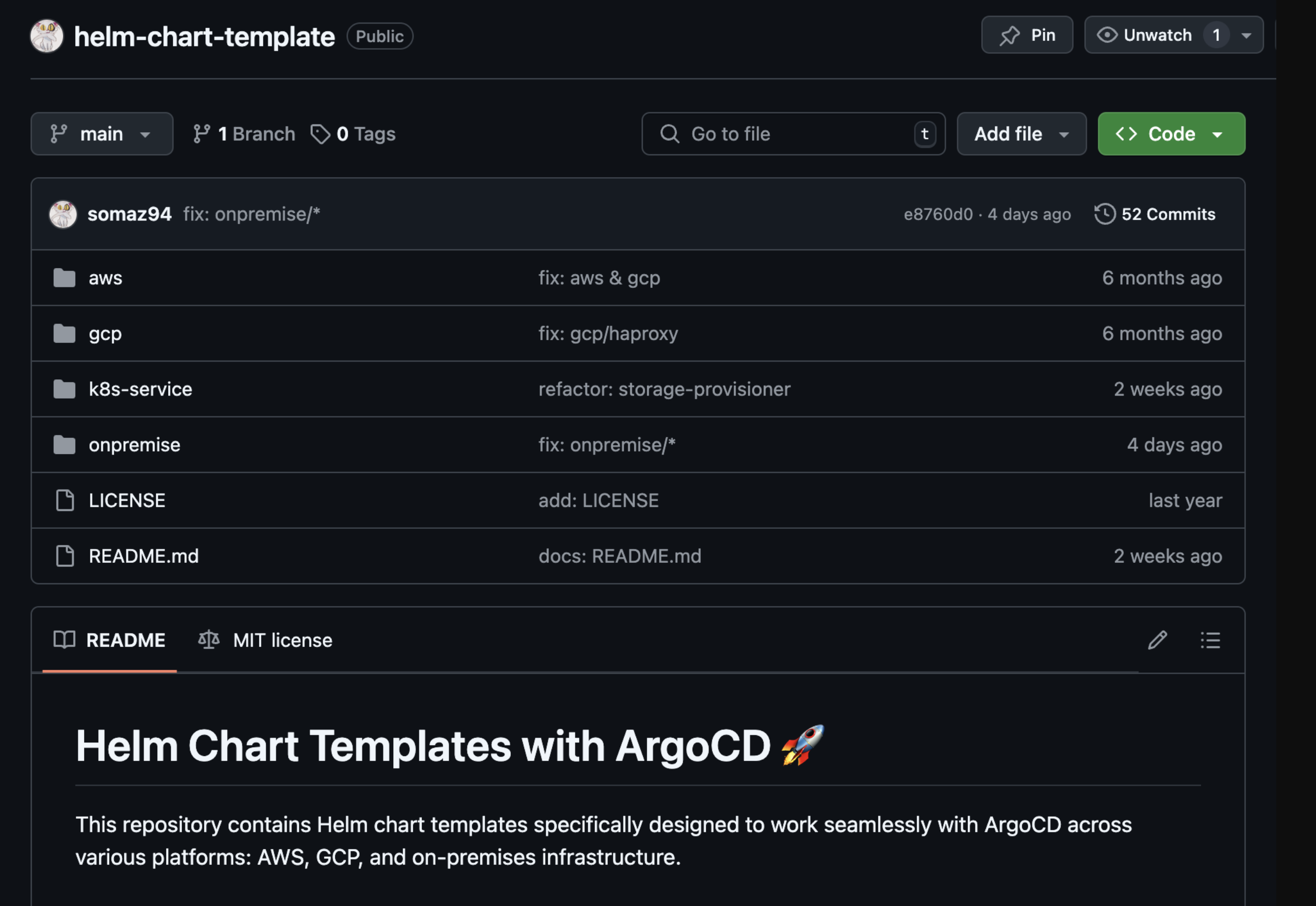
Overview
Helm Chart templates use a combination of YAML and Go templating to define configurations for Kubernetes resources. Let’s explore the key syntax patterns and their usage.
Helm Chart Template Syntax
Standard YAML Syntax
Basic YAML structure used in Helm charts:
apiVersion: v1
kind: Service
metadata:
name: my-service
labels:
app: my-app
Go Templating Syntax
Uses {{ ... }}
for dynamic values:
metadata:
name: {{ .Release.Name }}-service
- Values Reference (.Values)
- Accesses values.yaml configurations
- Example:
{{ .Values.replicaCount }}
- Release and Chart Metadata
- .Release: Release information
- .Chart: Chart metadata
- Example:
{{ .Chart.Name }}-{{ .Chart.Version }}
Control Structures
Conditional Statements
- Conditional statements allow sections to be rendered according to specified conditions
{{- if .Values.service.enabled }}
apiVersion: v1
kind: Service
metadata:
name: {{ .Release.Name }}-service
{{- end }}
Loops (range)
- Loops allow sections to be rendered multiple times
ports:
{{- range .Values.ports }}
- port: {{ . }}
{{- end }}
Functions and Operators
Default Values
- default provides an alternative value if the value is not set.
replicas: {{ .Values.replicaCount | default 1 }}
Pipeline Operators
- Pipeline operators allow you to chain functions together
host: {{ .Values.hostname | quote }}
Use Examples (pipeline)
host: "example.com"
YAML Conversion
- toYaml converts a value to YAML format
env:
{{ .Values.envVars | toYaml | indent 4 }}
Use Examples (toYaml)
envVars:
- name: ENV_VAR1
value: "value1"
- name: ENV_VAR2
value: "value2"```
Indentation and Nesting (indent, nindent)
- indent and nindent are used to add indentation to the output
- indent: Add a specified number of spaces before each line of text without adding a line break at the beginning.
- nindent: It's similar to indent, but the beginning includes a line break, which is useful for starting a new section within YAML.
Use Examples (nindent)
metadata:
labels:
{{- include "mychart.labels" . | nindent 6 }}
- nindent 6 adds 6 spaces at the beginning of each line in the output and aligns within labels, starting with a line break.
Use Examples (indent)
env:
{{ .Values.envVars | toYaml | indent 4 }}
- Indent the YAML structure of .Values.envVars by 4 spaces.
Include
include is used to include a template
{{- include "mychart.labels" . | nindent 4 }}
- Include the template mychart.labels and indent it by 4 spaces.
Use Examples (include)
metadata:
labels:
{{- include "mychart.labels" . | nindent 4 }}
Template Definitions and Usage
- Helm allows you to define reusable templates within a chart using define and template. (e.g.
_helpers.tpl
)
Defining Templates
{{- define "mychart.labels" -}}
app: {{ .Chart.Name }}
release: {{ .Release.Name }}
{{- end }}
Using Templates
metadata:
labels:
{{ include "mychart.labels" . | nindent 4 }}
Practical Example: Deployment Template
Here’s a comprehensive example of a deployment template:
Comments