3 min to read
Docker Layers - A Deep Dive into Dockerfile Build Principles
Exploring Docker image layers and build optimization techniques
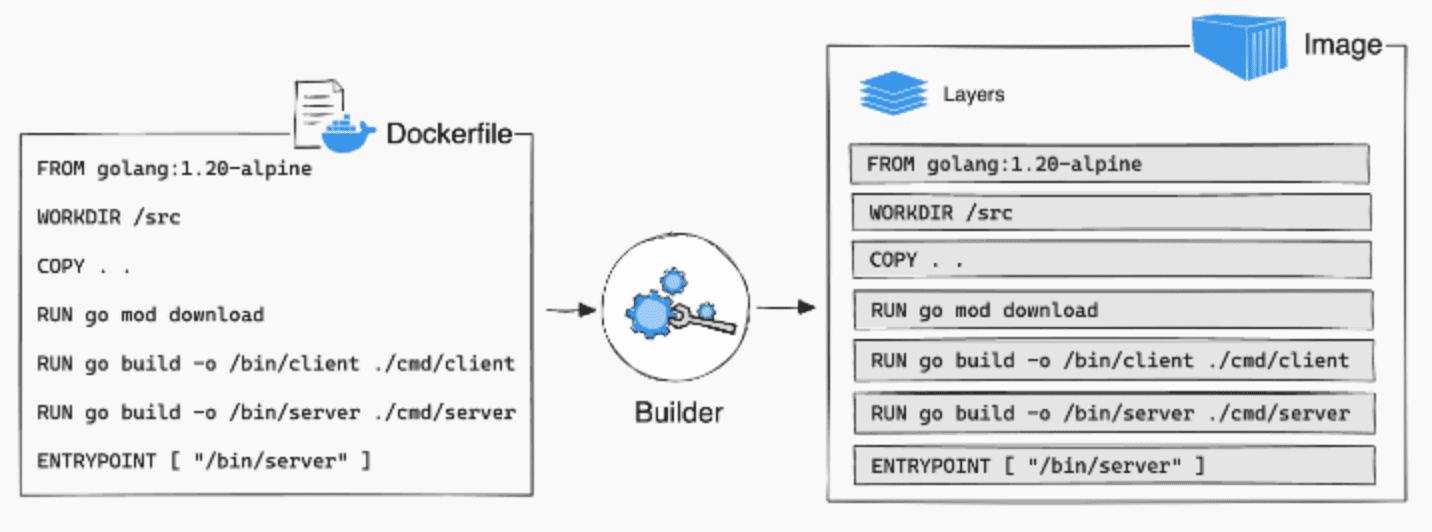
Overview
Following our previous discussion about Dockerfiles, today we’ll explore Docker layers and build principles in depth. Understanding these concepts is crucial for creating efficient and maintainable Docker images.
Dockerfile Build Principles
Dockerfile is a script that contains a series of instructions on how to build a Docker image.
Docker images consist of a series of layers, each of which represents Dockerfile’s instructions.
Understanding the building principle and layers is important in creating an efficient and easy-to-manage Docker image.
1. Base Image
Every Dockerfile starts with a base image specified using the FROM
instruction:
FROM ubuntu:latest
2. Instructions
Dockerfile contains several key instructions:
RUN
: Execute commands in shellCOPY/ADD
: Copy files from host to imageWORKDIR
: Set working directoryENV
: Set environment variablesEXPOSE
: Inform Docker about network portsCMD/ENTRYPOINT
: Specify container startup commands
3. Layers
Each instruction creates a new layer in the Docker image.
4. Caching
Docker optimizes builds by caching unchanged layers.
5. Final Image
The result is a cumulative stack of all layers.
Understanding Docker Layers
Docker images consist of read-only layers, each representing a Dockerfile instruction. Here’s how they work:
Layer Operation
- Created by the `FROM` instruction
- Contains OS or runtime environment
- Created by filesystem-modifying instructions
Example Dockerfile:
RUN apt-get update
COPY . /app
ENV PORT=8080
- Each layer is stored separately
- Only new layers are downloaded during pulls
Benefits of Layers
- Layers can be shared across images
- Saves disk space
- Improves download speed
- Cached layers are reused
- Faster build times
- Layers are read-only
- Ensures consistency
Layer Caching
Caching is a key feature of Docker layers:
# Good caching practice
COPY package.json package-lock.json ./
RUN npm install
COPY . .
vs
# Poor caching practice
COPY . .
RUN npm install
Examples
1. Standard Dockerfile
FROM ubuntu:18.04
RUN apt-get update && apt-get install -y python3
COPY . /app
WORKDIR /app
RUN pip3 install -r requirements.txt
CMD ["python3", "app.py"]
Layer breakdown:
- Ubuntu base image
- Python installation
- Application code
- Dependencies
- Command configuration
2. Multi-Stage Build
# Build stage
FROM node:12 as build
WORKDIR /app
COPY . .
RUN npm install && npm run build
# Production stage
FROM nginx:alpine
COPY --from=build /app/build /usr/share/nginx/html
Benefits:
- Smaller final image
- Separation of build and runtime environments
- Better security
Comments